Methods
Descriptions of the methods for the Kustomer Cards SDK.
This article describes the supported methods for cards and the supported methods for launching additional card-js
-based modals.
Kustomer.initialize
The Kustomer.initialize(options, callback)
method returns the context the user selects and views on the customer timeline or another UI element.
If the user collapses all items in the timeline, the context returns just the customer information.
If the user expands the conversation for a custom object (or KObject), the context will include data about the customer and the current timeline item.
See Context to learn more about the types of contexts you can return using the Kustomer Cards SDK.
Example:
window.Kustomer.initialize(
(context: Context, config: { userRoles: UserRoles }) => {
resolve({ ...context, ...config });
},
);
Sample Context Response
{
"type": "conversation",
"customer": {
"type": "customer",
"id": "57586826659e8bxxxxxxxxx",
"attributes": {
"displayName": "frank@kustomer.com",
"emails": [{
"email": "frank@kustomer.com",
"hash": "bb4aacbf457257d3839c00d96c0xxxxx",
"verified": false,
"type": "home",
"id": null
}],
"phones": [{
"phone": "+12125551212",
"hash": "f2988d112c897893d257b8f7de1xxxxx",
"verified": false,
"type": "mobile",
"id": null
}],
"socials": [],
"urls": [],
"locations": [{
"type": "home",
"name": "home",
"regionName": "New York",
"areaCode": "212",
"longitude": -74.0078,
"latitude": 40.733000000000004,
"zipCode": "10014",
"cityName": "New York",
"regionCode": "NY",
"countryName": "United States",
"countryCode": "US",
"id": null
}],
"activeUsers": ["57504b434c80120f00fxxxxx"],
"watchers": [],
"recentLocation": {
"updatedAt": "2016-08-04T21:44:46.482Z",
"location": {
"zipCode": "92129",
"regionName": "CA",
"cityName": "SAN DIEGO",
"countryName": "US",
"name": "home",
"type": "home",
"id": null
}
},
"createdAt": "2016-06-08T18:47:02.654Z",
"updatedAt": "2016-08-08T15:53:33.024Z",
"modifiedAt": "2016-07-28T18:34:11.576Z",
"lastActivityAt": "2016-08-04T21:44:46.508Z",
"lastMessageIn": {
"sentiment": {
"confidence": -0.193811,
"polarity": -1
}
},
"lastConversation": {
"tags": [],
"sentiment": {},
"id": "57a3b74eb1feb21100bf1335"
},
"conversationCounts": {
"done": 3,
"open": 1,
"snoozed": 0,
"all": 4
},
"preview": {
"text": "Automated summary of last conversation text",
"type": "message_in",
"previewAt": "2016-08-04T21:44:46.422Z"
},
"tags": [],
"progressiveStatus": "open",
"rev": 183
},
"relationships": {
"org": {
"links": {
"self": "/v1/orgs/57504b434c80120fxxxxx"
},
"data": {
"type": "org",
"id": "57504b434c80120f00xxxxx"
}
},
"messages": {
"links": {
"self": "/v1/customers/57586826659e8b10006xxxxx/messages"
}
},
"modifiedBy": {
"links": {
"self": "/v1/users/57504b434c80120f00fxxxxx"
},
"data": {
"type": "user",
"id": "57504b434c80120f00fxxxxx"
}
},
"company": {
"links": {
"self": "/v1/companies/5798ccb1366f7e11001xxxxx"
},
"data": {
"type": "company",
"id": "5798ccb1366f7e11001xxxxx"
}
},
"orders": {
"links": {
"self": "/v1/customers/57586826659e8b10006xxxxx/klasses/orders"
},
"meta": {
"kobject": true
}
}
},
"links": {
"self": "/v1/customers/57586826659e8b10006xxxxx"
}
},
"conversation": {
"type": "conversation",
"id": "57a3b74eb1feb21100bxxxxx",
"attributes": {
"name": "Subject of conversation",
"preview": "Automated summary of last conversation text",
"channels": ["email"],
"status": "open",
"messageCount": 1,
"noteCount": 0,
"satisfaction": 0,
"createdAt": "2016-08-04T21:44:46.441Z",
"updatedAt": "2016-08-04T21:44:46.500Z",
"tags": [],
"suggestedTags": [],
"sentiment": {},
"lastMessageIn": {
"id": "57a3b74d9a8aad11006xxxxx",
"sentAt": "2016-08-04T21:44:46.422Z"
},
"assignedUsers": []
},
"relationships": {
"org": {
"links": {
"self": "/v1/orgs/57504b434c80120f00fxxxxx"
},
"data": {
"type": "org",
"id": "57504b434c80120f00fxxxxx"
}
},
"customer": {
"links": {
"self": "/v1/customers/57586826659e8b10006xxxxx"
},
"data": {
"type": "customer",
"id": "57586826659e8b10006xxxxx"
}
},
"messages": {
"links": {
"self": "/v1/conversations/57a3b74eb1feb21100bxxxx/messages"
}
}
},
"links": {
"self": "/v1/conversations/57a3b74eb1feb21100xxxxx"
}
}
}
Sample Config Response
{
"userRoles": {
"org.admin": false,
}
}
Kustomer.request
The Kustomer.request(options, callback)
method allows the card to make an API request with the permissions of the user. You can use the request method to retrieve additional information, update an existing record, create a new record, or insert a draft response.
Example:
In the example below, the user is requesting to change the status of a conversation to "Done."
Kustomer.request(options, callback)
The options object currently supports:
{
url?: string; // The API endpoint, such as ‘/v1/customers’
method: string; // The API call method. Either ‘GET’, ‘POST’, ‘PUT’, ‘PATCH’, or ‘DELETE’
options?: Record<string, any>;
body?: Record<string, any>; // The request body
modalToken?: string; // A token passed from the front-end to verify you are targeting the correct modal
targetModal?: boolean; // Specifies whether or not a request will also be sent to a currently visible modal
}
const varConversationId = 57a3b74eb1feb21100bf1335;
Kustomer.request({
url: '/v1/conversations/' + varConversationId,
method: 'put',
body: {"status": "done"}
}, function(err, conversations) {
if (err || !conversations ) {
console.log(err);
return;
}
});
Kustomer.close
The Kustomer.close()
method removes the iFrame from the page if the iFrame is no longer relevant for the user.
Kustomer.open
The Kustomer.open()
method opens the app widget iFrame.
Kustomer.openCustomer
The Kustomer.openCustomer('customer-id')
method opens the specified customer timeline.
Kustomer.openCustomerEvent
The Kustomer.openCustomerEvent('customer-id', 'event-id')
method opens the specified customer timeline to the specified event.
Kustomer.resize
The Kustomer.resize(options)
method receives an optional object that updates the height or width of the card or of the modal.
If no height is provided, the card dynamically resizes to the height of the page in the card.
Optionally, you can pass an explicit height. The height should be a string such as '100px'.
The possible options for resize
are:
{
height?: string; // Specifies the height you wish the card to resize to. If the height not passed, the height is generated dynamically
width?: string; // Specifies the width you wish the card to resize to. If the width is not passed, the width will not change and the width will not be generated dynamically.
modal?: boolean // Specifies if the resize event is targeting a card, or the associated modal.
}
Kustomer.updateStatus(params)
The params
object currently supports
{
status: 'info' | 'warning' | 'danger';
description: string; // a friendly message to your users about the current state of the kview
}
Call the Kustomer.updateStatus
method to supply the kview with an informational banner, notifying the user of the current state of the kview. This can be useful when network connections to 3rd parties are failing, or if your kview app detects any instability that you want to message that to the user. The three supported status
strings above all map to differently-colored banners, to help relay the level of status change one might be experiencing.
Kustomer.clearStatus
Call the Kustomer.clearStatus()method to remove any status notifications provided by
Kustomer.statusChange`.
Kustomer.handleTriggerToast(params)
Call the Kustomer.handleTriggerToast(params)
method to trigger toast notifications when you want to give feedback to your users.
The params
object currently supports
{
type: 'info' | 'warning' | 'error' | 'success';
message: string; // A friendly message to your users as feedback of something that happened in your app
}
Kustomer.expandInsightsCard(params)
Call the Kustomer.expandInsightsCard(params)
method to expand a view that takes up the entire insights panel. This view will persist as agents are navigating between events and conversations within the same timeline and will automatically contract when an agent navigates to a new customer's timeline.
The params
object currently supports
{
appName: string; // Your app name
appLogo: string; // App logo URL
title: string; // Expanded insights card title
height: number; // Height of expanded insights card
url: string; // URL to be iframed in the view
context: any; // Data to be passed into the expanded insights card view
}
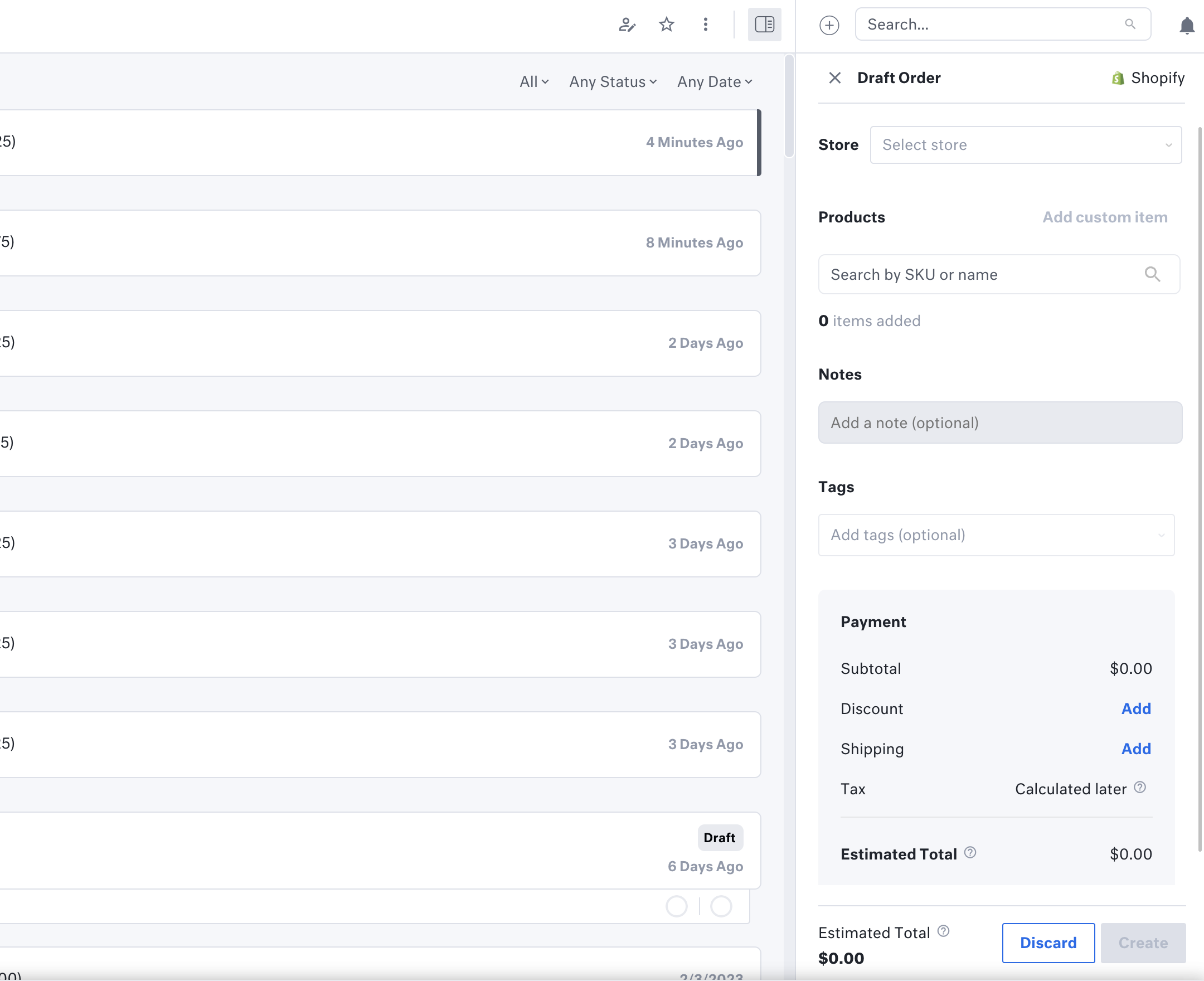
Kustomer.contractInsightsCard
Call the Kustomer.contractInsightsCard()
method to close the expanded insights card programmatically.
Kustomer.on
Call the Kustomer.on
method to subscribe to several supported events in the SDK. The supported events are:
context
context
Kustomer.on('context', function(context) {
// Takes action on the context change. Uses a new context object similar to the above sample.
});
Response Object
{
'subscribed-feature-flag-1': true,
'subscribed-feature-flag-2': false,
}
number-dialed
number-dialed
Kustomer.on('number-dialed', function(context) {
// Takes action if the number-dialed has changed
});
Response Object
{
}
routing-status-change
routing-status-change
Kustomer.on('routing-status-change', function(context) {
// Takes action if a routing-status-change has changed
});
Response Object
{
}
Updated over 2 years ago