Voice / VoIP apps
A developer guide for widget-based Voice / VoIP apps with status syncing and click-to-dial functionality.
We'd love to get your feedback!
We want our docs to be clear, accurate, and usable. Create and log in to your ReadMe account to suggest edits, ask questions, and provide feedback.
In this guide, we'll show you app components you can use to build a widget-based Voice or VoIP app integration on the Kustomer Apps Platform with the following features:
- Click-to-dial functionality
- Agent status syncs between Kustomer and a Voice / VoIP platform
Resources
This guide discusses app features and components common for Voice or VoIP apps built on the Kustomer Apps Platform. For an introduction to these components, we recommend the following sections from our apps platform documentation:
Introduction section
What types of apps can I build?
App Object Model section
Advanced section
Sample app definition
We've provided a sample app definition for a public Voice / VoIP app with status syncing and click-to-dial functionality.
App definition highlights
We've highlighted app definition properties that are important to consider for Voice / VoIP apps built on the Kustomer Apps Platform.
-
meta.exernalQueue
: Required to connect a queue for the external Voice / VoIP app and to route events to the app. -
dataSubscriptions
: Required to connect your app to events published by Kustomer. -
settingsPageConfig
: Required to load an iFrame-based custom settings page that allows Admins to map a status in Kustomer to a status in the Voice / VoIP platform. -
widgets
: Required to load an iFrame-based Voice / VOIP widget to run within Kustomer.
Sample app defintion for Sync, Inc
Our sample app definition references a fictional Voice / VoIP platform called Sync, Inc in the following property definitions:
appDetails.externalPlatform
,meta.externalQueue
, andsettingsPageConfig
.
{
"app": "status_sync_example_app",
"version": "0.0.1",
"visibility": "public",
"title": "Status Syncing Sample App",
"description": "A sample Voice / VoIP app with status syncing that features bidirectional communication for agent statuses and click-to-dial functionality.",
"appDetails": {
"appDeveloper": {
"name": "Kustomer",
"website": "https://kustomer.com",
"supportEmail": "[email protected]"
},
"externalPlatform": {
"name": "Sync, Inc",
"website": "https://www.example.com"
},
"meta": {
"externalQueue": "sync_inc",
},
"dataSubscriptions": [
"click-to-dial",
"status-sync"
],
"settingsPageConfig": {
"title": "Sync, Inc",
"url": "<<A link to your externally hosted custom settings page. This custom settings page is needed to connect statuses in Kustomer to statuses in the Voice / VoIP platform.>>",
"description": "A sample custom settings page that renders in an iFrame within the Kustomer settings page. This custom settings page allows Admins to map a status in Kustomer to a status in the Voice / VoIP platform.",
},
"widgets": [{
"url": "mywidget.com",
"icon": "mywidget.com/icon.png",
"height": "600",
"width": "300",
}]
}
Map agent status with a Custom Settings Page
You can use a Custom Settings Page to map connections between agent statuses in Kustomer and your selected Voice / VoIP platform.
The Custom Settings Page loads within an iFrame and allows you to map the connections needed to sync agent statuses between Kustomer and the Voice / VoIP platform.
Fetch, map, and update agent statuses
Configure the Custom Settings Page iFrame to fetch and update the external status map to the agent statuses defined in Kustomer. The configuration requires you to do the following:
- Fetch configured agent statuses during app initialization
- Map and represent configured agent statuses
- Update the mapping for configured agent statuses
Fetch configured agent statuses during app initialization
To collect the currently configured statuses for a given Kustomer organization, make a GET request to the /v1/routing/statuses
endpoint when you initialize the Cards SDK in your app.
We've included a code sample with a sample request below.
Initialize the Cards SDK to make API calls between Kustomer and an embedded application
Use
Kustomer.initialize
to initialize the Cards SDK and to allow your embedded external application to communicate with Kustomer.
Sample request: Fetch configured agent statuses
export function request<T>(params) {
return new Promise((resolve, reject) => {
window.Kustomer.request(params, (err, response) => {
if (err) {
return reject(err);
}
return resolve(response);
});
});
}
export const fetchKustomerStatuses = () => {
const params = {
method: 'GET',
url: '/v1/routing/statuses',
};
return request(params);
};
Map and represent configured agent statuses
After you fetch the possible configured agent statuses from Kustomer, you can map these to configured statuses within the Voice / VoIP platform.
One way to represent this agent status mapping is to use a set of dropdown lists to connect a single status within Kustomer to an externally defined status.
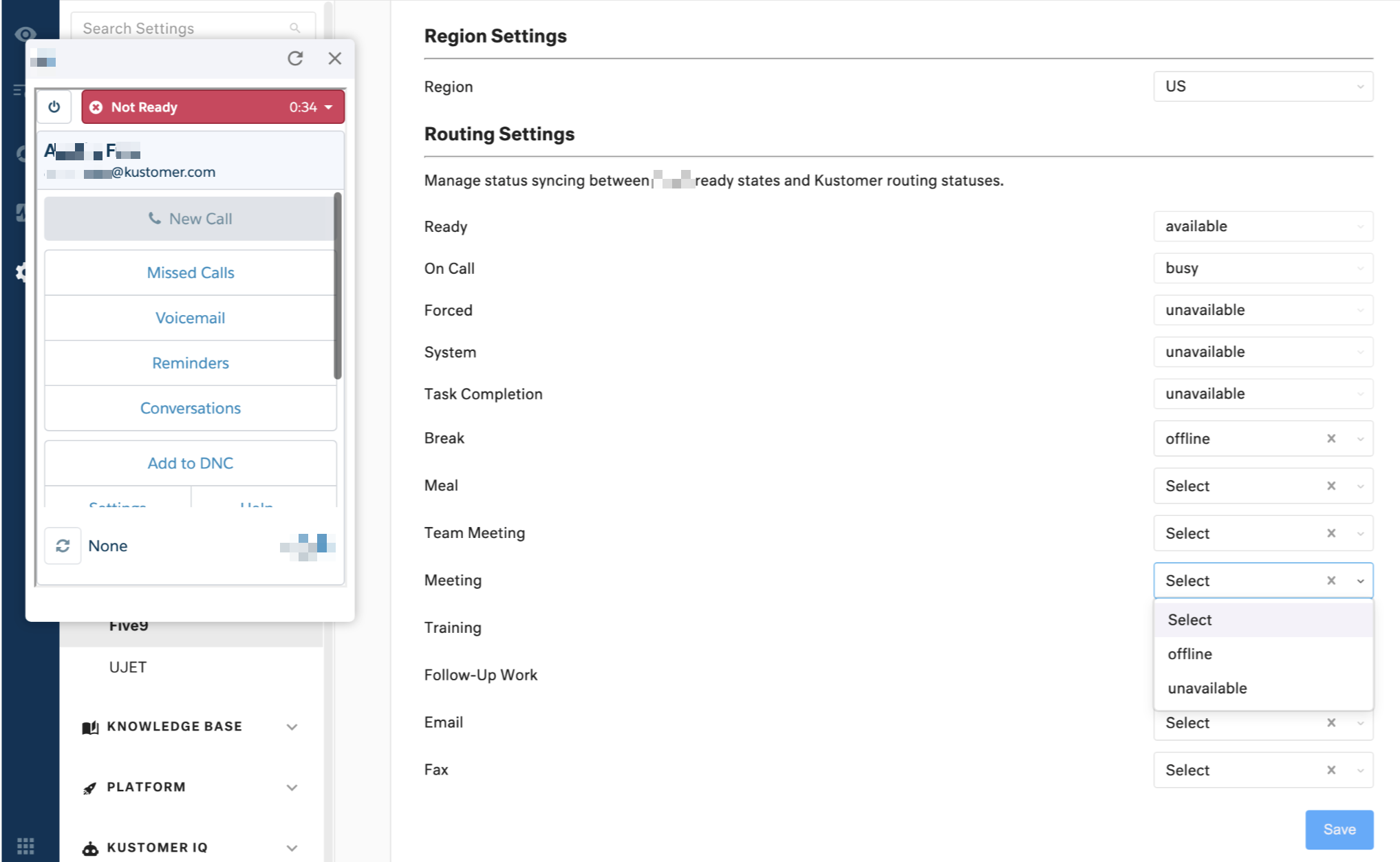
Custom settings page with a set of dropdown lists to connect statuses within Kustomer to externally defined statuses.
Update the mapping for configured agent statuses
To update the mapping for a configured agent status, make a PUT request to the /v1/routing/statuses/${kustomerStatusId}
endpoint with your given mapped status ID.
Sample request: Update configured agent statuses
export const mapKustomerStatusToExternalStatus = (kustomerStatusId, existingMaps, statusId) => {
const params = {
method: 'PUT',
url: `/v1/routing/statuses/${kustomerStatusId}`,
body: {
externalStatusMap: {
...existingMaps, // relevant for any other configured statuses
sync_inc: statusId,
},
},
};
return request(params);
};
Subscribe to events
The Kustomer Cards SDK supports the following statuses you can use to subscribe to events in your widget-based Voice / VoIP app: number-dialed
, routing-status-change
, and fallbackStatusType
.
Listen for agent status changes in Kustomer
To interact with your selected Voice / VoIP platform when something changes within Kustomer, use the number-dialed
and routing-status-change
data subscriptions with the Kustomer.on
method in the code for the application embedded in the widget.
Update agent statuses in Kustomer
To allow an app to update agent statuses within Kustomer, use fallbackStatusType
with the Kustomer.requestUpdateUserStatus
method in the code for the application embedded in the widget.
number-dialed
Subscribe to number-dialed
for the Cards SDK to send an event to provide the phone number for a call:
Sample code: number-dialed
event
window.Kustomer.on('number-dialed', (number) => this.dialNumber(number));
routing-status-change
Subscribe to routing-status-change
to listen for changes to an agent's status when Kustomer routes events to them (for example, calls, messages, and so on).
Sample code: routing-status-change
event
window.Kustomer.on('routing-status-change', (statusData) =>
this.handleKustomerStatusChange(statusData));
fallbackStatusType
Use the Kustomer.requestUpdateUserStatus
method with fallbackStatusType
to allow an app to update and set agent statuses in Kustomer based on events from within the external Voice / VoIP platform.
Sample code: fallbackStatusType
event
window.Kustomer.requestUpdateUserStatus({ fallbackStatusType: 'busy' });
Create an external queue
The meta.externalQueue
property allows you to specify an external queue name for the voice channel app.
meta.externalQueue
Use externalQueue
on the meta
property to assign voice channel teams to the external queue and to exclude voice app calls from routing to other teams.
Once configured, the external queue name appears as an option in Kustomer under Settings > Users > Teams > [Voice channel team name] > Routing Profile in the External Queues block.
Sample app definition snippet
...
"meta": {
"externalQueue": "sync_inc"
},
...
Updated about 3 years ago